R tidyverse: readr
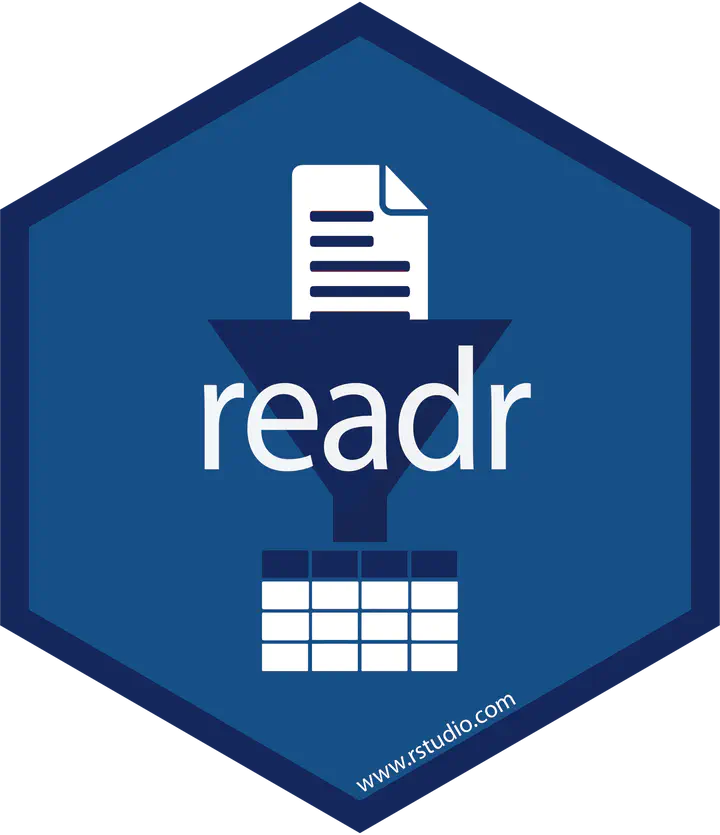
Many times you have to import you own data instead to write it directly from , especially if you work with spreadsheets, manage data and work with people who does not know to use , or any other programming language yet.
Import data with readr
This package is really popular to import tabular data, especially .csv files, these are the most common function in readr
package.
-
read_csv() Import comma separate files
-
read_csv2() Import semicolon separate files
-
read_delim() Import any file with a delimiter (obviously specifying that character)
library(tidyverse)
library(readr)
# readr package is inside tidyverse package
# we are just calling it explicitly but it is not necessary
x <- read_csv("~/Documentos/R/Rdummies/Pesos_familia.csv")
head(x) # Show the first 6 lines in our document
x <- read_csv("~/Documentos/R/Rdummies/Pesos_familia.csv")
head(x)
Individuo | Mes | Peso | Mes_ |
---|---|---|---|
Sara | 3 | 63.6 | 01/03/20 |
Sara | 4 | 64.1 | 01/04/20 |
Sara | 5 | 62.2 | 01/05/20 |
Sara | 6 | 62.5 | 01/06/20 |
Sara | 7 | 62.6 | 01/07/20 |
Sara | 8 | 61.9 | 01/08/20 |
If you want to import Excel files is better user readxl
package, you could download the next dataset a work by yourself.
Download example_dataset.xlsx.
library(readxl)
My_dataset <- read_excel("~/Documentos/R/R_personal_website_2.0/static/uploads/example_dataset.xlsx",sheet = 1,col_names = T)
# You must change path
# if you ar using windows you must use "\\" instead "/"
head(My_dataset[,1:4]) # we are showing the firts four colums for example purpose
## # A tibble: 6 × 4
## tipo_doc nume_doc nom1 nom2
## <chr> <chr> <chr> <chr>
## 1 CC 40733306 NINI JOHANNA
## 2 CC 1010039739 BRAYAN MAURICIO
## 3 CC 28826543 MARIA ANTONIA
## 4 CC 28752527 MARIA ANGELA
## 5 TI 1109520069 LEXAIR ATONIO
## 6 CC 28567101 MARIA EDITH
parsing a vector
To evalaluate an object in you can use str() o class() but to parse a vector you should use parce_() functions
str(parse_logical(c("TRUE", "FALSE", "NA")))
## logi [1:3] TRUE FALSE NA
str(parse_integer(c("1", "2", "3")))
## int [1:3] 1 2 3
str(parse_date(c("2010-01-01", "1979-10-14")))
## Date[1:2], format: "2010-01-01" "1979-10-14"
parse_integer(c("1", "231", ".", "456"), na = ".")
## [1] 1 231 NA 456
Then, when the parsing fails, you will get a warning
x <- parse_integer(c("123", "345", "abc", "123.45"))
## Warning: 2 parsing failures.
## row col expected actual
## 3 -- no trailing characters abc
## 4 -- no trailing characters 123.45
If you want a detail report about problems in a vector you should use problems() function to create a tibble
x <-parse_number(c("123", "345", "abc", "123.45"))
## Warning: 1 parsing failure.
## row col expected actual
## 3 -- a number abc
x <- problems(x)
x
## # A tibble: 1 × 4
## row col expected actual
## <int> <int> <chr> <chr>
## 1 3 NA a number abc